Enhancing Platform Pages with Web Extensions
So you want to build an amazing user experience in Skedulo? Platform pages will give you most of what you need, but what if you need to go just that little bit further?
Perhaps you need a completely custom component that doesn’t yet exist in the component library, for example you want to embed a map that can show an address based on url parameters in your platform page. Or maybe you want to leverage some of your existing web extensions within your shiny new platform pages.
Well, enter our old friend, web extensions. Did you know, you can embed a web extension inside of a platform page? Not only that, you can pass data to it in a number of ways!
Before we begin, this blog will cover embedding and passing data to a custom web extensions from within platform pages, and assumes you are familiar with both the development of web extensions and platform pages.
Let’s start with the Web Extension
The first thing you need to know is; your web extension needs to be part of a package for this to work, so if it’s a standalone extension you will need to redeploy it as part of a package.
With that out of the way, we will take a look at the web extension part first.
Web extensions have a number of injected variables you can use to interact with the Skedulo Pulse Platform, the one we are concerned with today is the params variable.
Normally, this is used to get the URL parameters provided to your web extension, and that is how we will use it here, but with a twist.
Here is an example of a very simple map component, that simply takes an address URL parameter and provides an iframe’d google map for it.
import * as React from 'react'
import {context, params} from './Services/Services'
interface AppState {
data: any,
context: any,
params: any
}
export class App extends React.PureComponent<{}, AppState> {
constructor(props: {}) {
super(props)
this.state = {
data: {},
context: context,
params: params
}
}
async componentDidMount() {
const parsedParams = JSON.parse(JSON.stringify(params));
if(parsedParams.address) {
this.setState({ data: { address: parsedParams.address } });
}
}
render() {
return (
<div className="App">
<iframe className="map-box"
height="1000"
width="100%"
src={`[https://www.google.com/maps/embed/v1/place?key=apikey&q=${this.state.data.address}`](https://www.google.com/maps/embed/v1/place?key=AIzaSyCYSwhlik5hY55X1fRTaxLy_cGN5nC963M&q=${this.state.data.address}`)}
>
</iframe>
</div>
)
}
}
Once you’ve got a web extension that handles URL parameters, let’s now go back to platform pages to see how we can interact with it.
Embedding the web extension in a platform page
Within your platform page, you want to add the tag. This tag will let you embed your web extension directly into the platform page.
Here is an example of this:
<platform-component
package-name="skedulo-web-extension"
name="WebExtension"
pkg-name="mappackage"
page-url="map"
context-a="C"
reference-uid="{{ _.queryParams.address }}">
</platform-component>
Looking at the properties for this component, we have package-name and name, these both tell the component that we want to embed a web extension.
Next we have pkg-name
and page-url
the first one refers to the package name (as displayed in the packages menu or the SDK) and the second one refers to the actual name of the web extension specified in the SDK.
The property context-a
is always set to C and finally we have the most important property;
reference-uid
This is what lets us pass information into the web extension.
Now normally, the value of the param variable in a web extension is whatever is passed in when it is accessed directly via its URL
https://mwheeler2-dev.my.skedulo.com/c/g/map?address=sw11aa
But, because we are embedding the web extension in a platform page, we need to first pass data from the platform page, into the web extension.
This is where reference-uid
comes in. Obviously, you could use this to pass in the UID
of a record, for example the one that is opened in the platform page and then use the data services in your web extension to look up the records details.
However, despite its name, you can actually pass in any string you like, and that string will be provided to the web extension as if it was in it’s URL, via its injected params variable!
Taking our example map web extension, if you wanted to pass in the address from the platform page’s url, it might look something like this (note how it’s now /platform/page/map
rather than /c/g/map
)
https://my-tenant.my.skedulo.com/platform/page/map?address=sw11aa
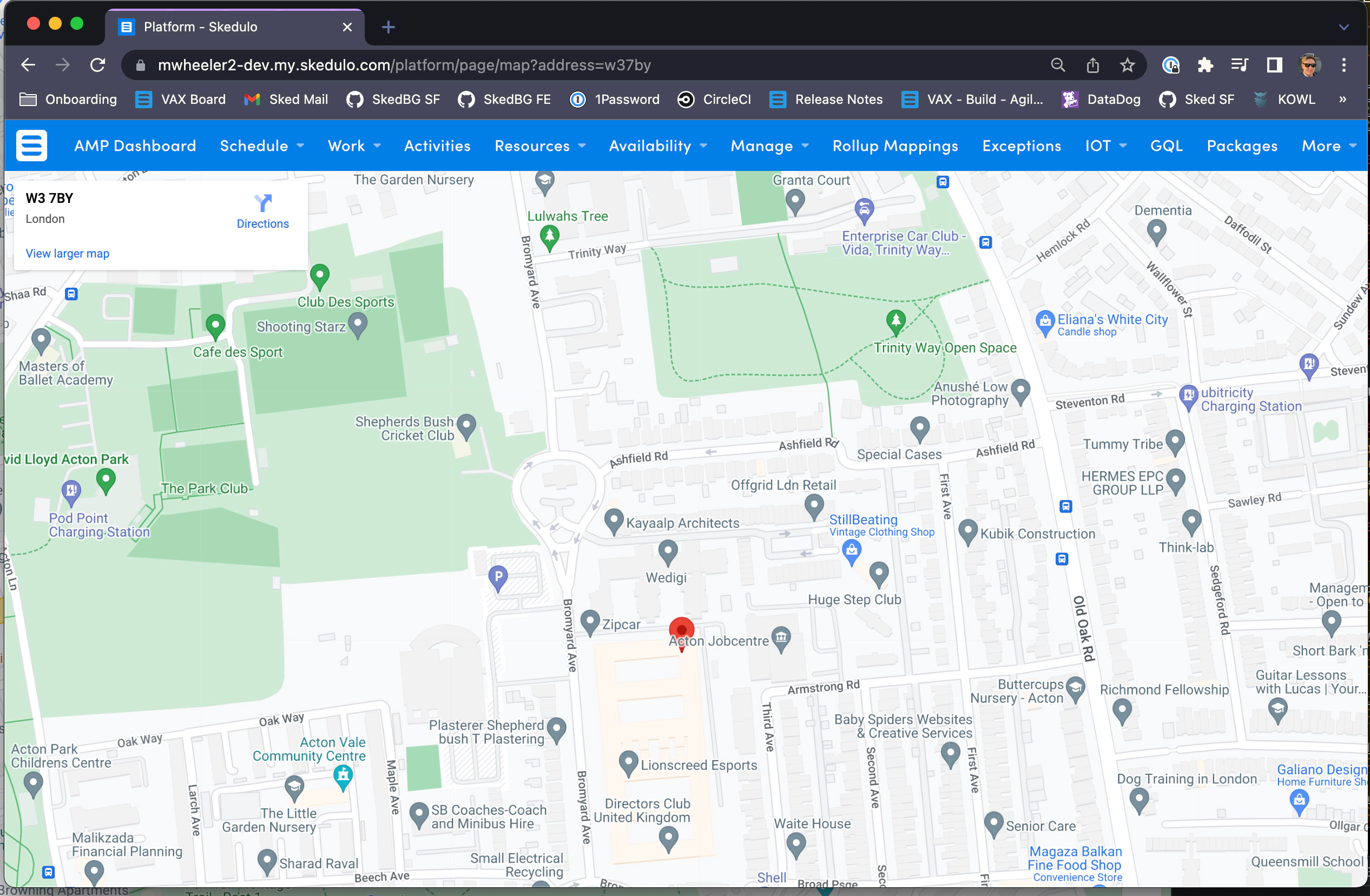
Now because we’re passing that value from the platform page, to the web extension via the reference-uid
property, the web extension now has access to an object with a key of “address” and a value of “sw11aa” which, just like it would if you were accessing it directly!
You can use multiple URL
parameters as needed, as well as pass multiple values to a single parameter.
For example:
reference-uid=”{{ _.queryParams.address }}&{{ _.queryParams.firstname }}”
Would look like /map?address=foo&firstname=bar
to your web extension, and you’d have both address
and firstname
keys within the params object.
And going to:
https://my-tenant.my.skedulo.com/platform/page/map?address=foo,bar
Would result in address being equal to foo,bar
Now you know how to enhance your platform pages with web extensions! We’d love to see what you come up with, or if you have any questions you can reach out to us on twitter @SkeduloDevs